Simple JavaFX project won't run with IntelliJ
This post is part the 100 Days of JavaFX Series.
If you try to run a simple JavaFX project like the one I used for my “Native GUI app with JavaFX” article in IntelliJ, you will run an error that looks like this:
Exception in Application start method
(...)
Caused by:
java.lang.IllegalAccessError: superclass access check failed: class com.sun.javafx.scene.control.ControlHelper (in unnamed module @0x183c1801) cannot access class com.sun.javafx.scene.layout.RegionHelper (in module javafx.graphics) because module javafx.graphics does not export com.sun.javafx.scene.layout to unnamed module @0x183c1801
Yet if you run the project using the mvn javafx:run
command it will work just fine:
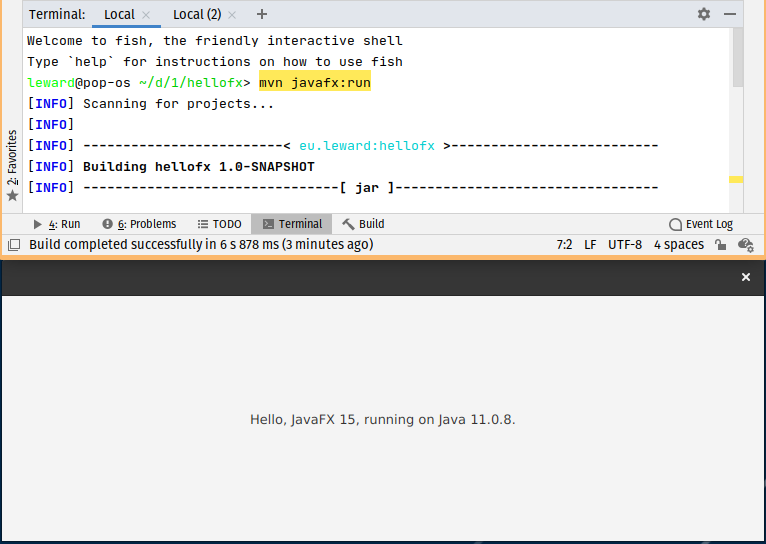
It looks like IntelliJ is not configuring the modules/classpath correctly out of the box.
A way to remediate this is to provide a module descriptor (module-info.java
):
// hellofx/src/main/java/module-info.java
module eu.leward.hellofx {
requires javafx.controls;
opens eu.leward.hellofx to javafx.graphics;
}
You won’t need to add a require
for [javafx.graphics](http://javafx.graphics)
and javafx.base
since those are transitive requirements from javafx.controls.
Now, you can click on the “Run” button for HelloFX
in Intellij and the application will start as expected.
Quick reminder on modules:
module eu.leward.hellofx
indicates the name of the module, I decided to follow a naming convention, but it can be any name you wantmodule toto
will work too even though it may not be the best nameopens eu.leward.hellofx to javafx.graphics;
hereeu.leward.hellofx
is a Java package name, not the name of module!opens to
is used becausemain
uses thelaunch()
method which does some refection to instantiate and run the application.
Using Java modules, you need to allow that reflection access usingopens
. You won’t get this error if you use classpath instead of modulepath, however I believe modules and modulepath is the way forward.
Maven Compiler Plugin
If you don’t make any modification to you pom.xml
, running mvn compile
will now result in an error:
Compilation failure
[ERROR] /helofx/src/main/java/module-info.java:[2,20] module not found: javafx.controls
If you specify anything, maven will use by default an old version of the maven-compiler-plugin
. So you need to specify a more recent version (>= 3.7.0) for compilation with modules to work:
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
</plugin>
</plugins>
With that out of the way, happy building and compiling!